User passkey management
Allow users to manage the passkeys associated with their account.
Get started by choosing your client and backend for personalized docs.
Select client
Select backend
Prerequisites
Complete the steps in Quickstart to create a Passage app and install the necessary SDKs.
Display passkey table
Fetch user devices from Passage
Retrieve the list of passkey devices for each user from the Passkey Flex Backend.
Use the unique external ID created during passkey registration to retrieve a user’s passkey devices from the Passage database. Learn more about external identifier.
Backend
app.get('/user/devices', async (req: Request, res: Response) => {
// ... Verify user identity
let user = User.findOne({id: req.session.id})
let passkeys: WebAuthnDevices [] = [];
// Verify that the Passage ID you created and stored is present
// This indicates that the user has passkeys
if(user.passageExternalId !== null) {
// Retrieve a list of all devices used to register a passkey
passkeys = await passage.getDevices(user.passageExternalId!);
}
return res.status(200).json({username, passkeys}).end();
});
Learn more about the Node.js Flex SDK
Display devices
After retrieving the list of devices, WebAuthnDevice
data can be rendered.
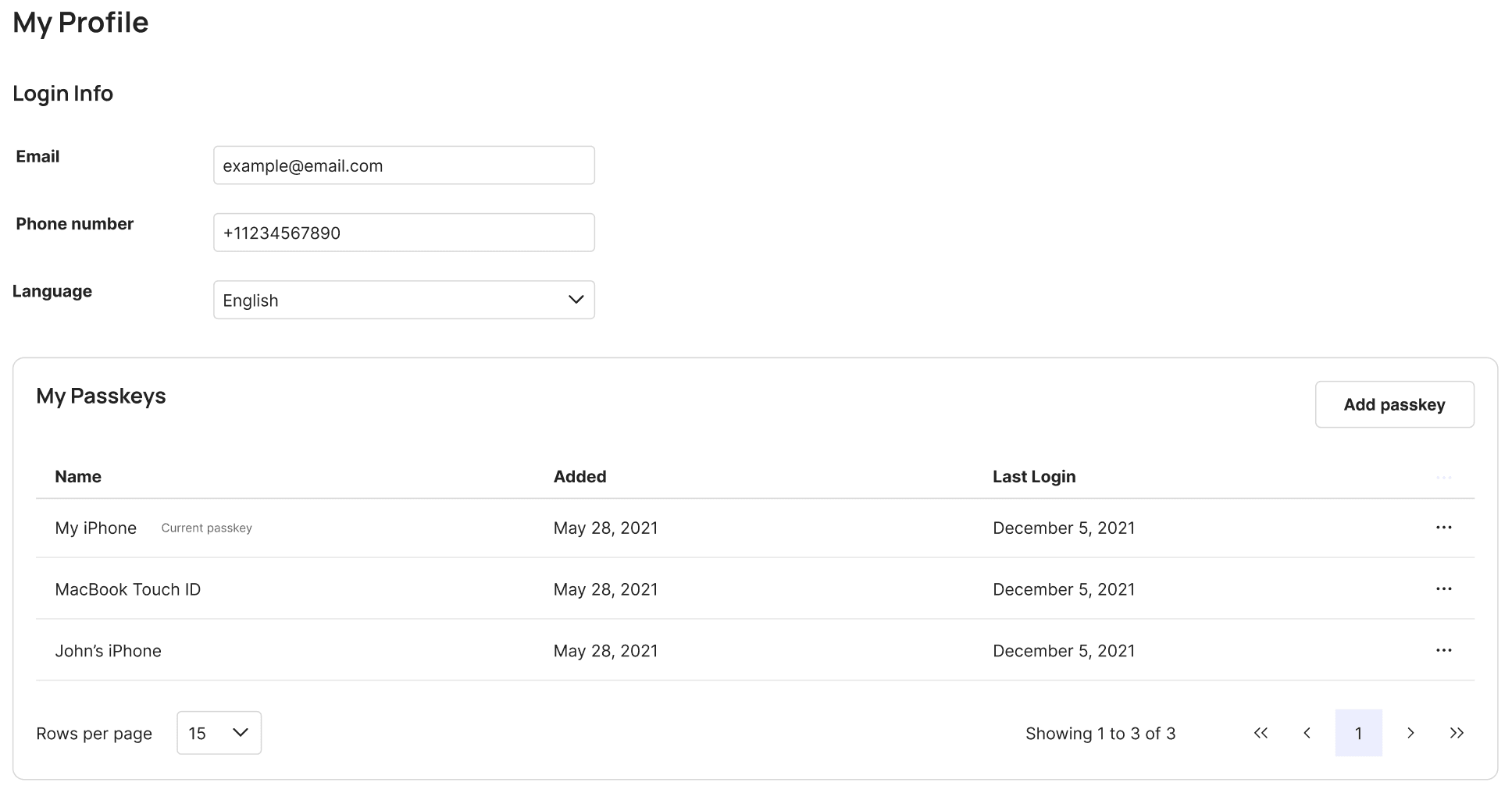
WebAuthnDevice properties
Property | Data Type | Description |
---|---|---|
createdAt | Date | Date the passkey was created. |
credId | string | WebAuthn credential id. |
friendlyName | string | Name set when the passkey was created. |
icons | WebAuthnIcons | Icon associated with the passkey: light and dark . |
id | string | Passkey unique identifier. |
lastLoginAt | Date | Date the passkey was last used to log in. |
type | WebAuthnType | The type of WebAuthn authenticator: passkey , security_key , or platform . |
updatedAt | Date | Date the passkey was last updated. |
usageCount | number | Number of times the passkey has been used. |
Revoke passkey
You can revoke a passkey from your server with the Passkey Flex backend SDK.
Backend
app.post('/revokePasskey', async (req: Request, res: Response) => {
// ... Verify user identity
let user = User.findOne({id: req.session.id})
// Revoke passkey device
await passage.revokeDevice(
user.passageExternalId! // Same value saved to your DB
req.body.id // ID of the passkey
);
return res.status(200).send('OK').end();
});