Custom Registration Data
Add fields to your registration page - no code required.
Adding custom fields to your registration page is simple with Passage. Learn how to define your custom registration page, allow users to update their own profile data, and manage user data from your web application.
Adding User Fields
You can define the fields you want on your registration page in the Passage Console. Define a new field by giving it a display name and a type. The currently supported types are:
- String
- Date (MM/DD/YYYY)
- Number
- Boolean (renders as a checkbox)
- Phone number
Email and phone number types are strings that will have additional validation performed to ensure they are valid email addresses or phone numbers.
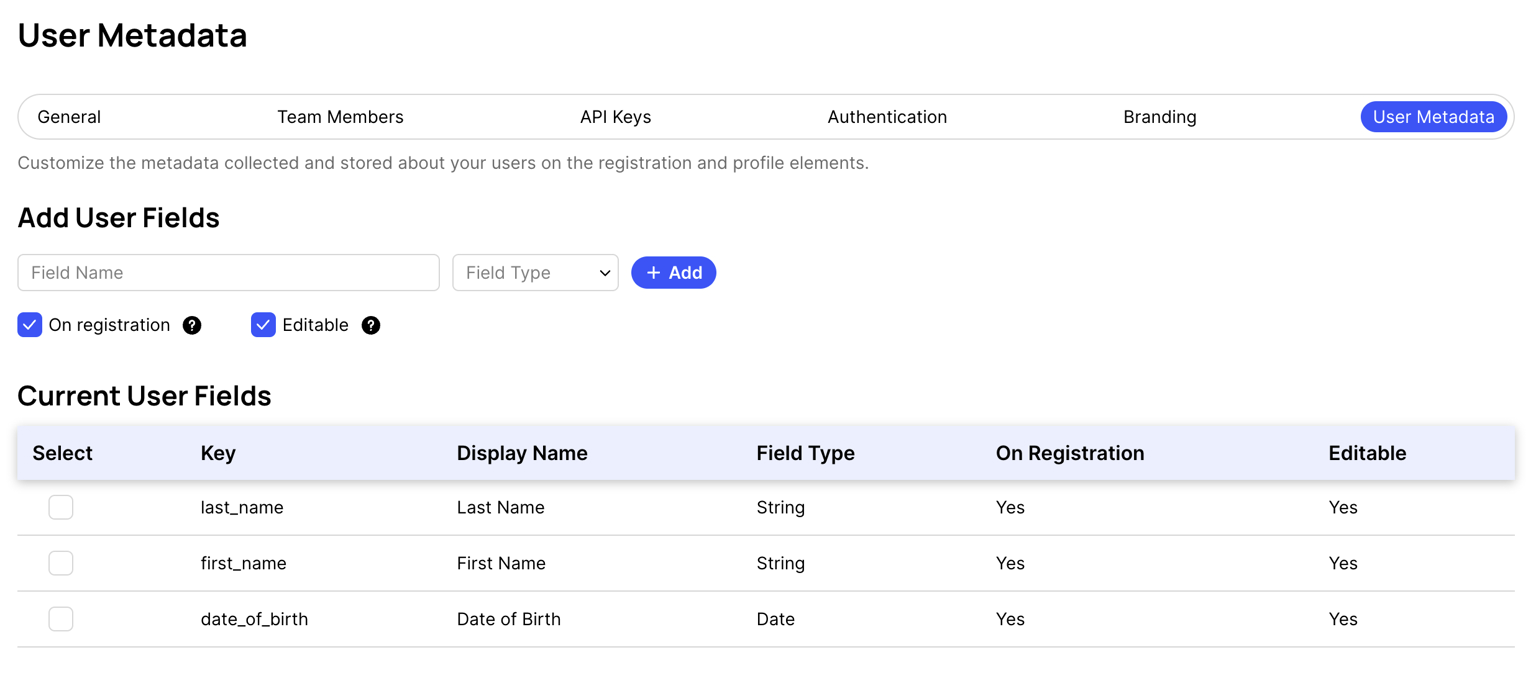
Fields also have a few settings associated with them to determine who can view and edit the fields:
- On Registration: if checked, the field will show up on the register element by default and be required for new users.
- Editable: if checked, the field will show up on the profile element by default and can be updated by a user via the Element or Passage-JS.
Fields not marked as editable, will only be accessible via the Management API.
Use the designer console to resize and reorder the fields for your Register and Profile Elements. Once saved, these changes will immediately be reflected in your application.
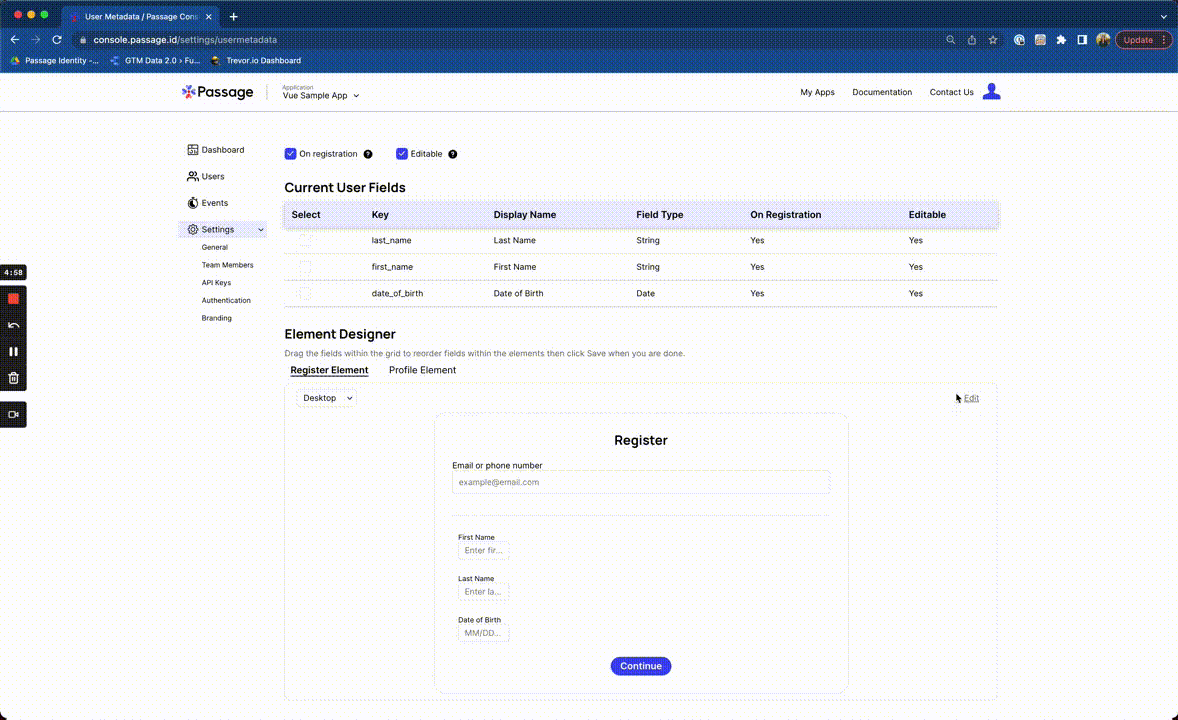
Access Fields on the User Model
Once you’ve defined the schema for your custom user fields, you can access those user attributes via the Passage Management API and backend SDKs. All user objects will contain a user_metadata
key which consists of a dictionary of field name and values based on the schema defined. If no metadata schema is defined, the user_metadata
key will be {}
. An example user response would look like this:
API
GET /v1/apps/:appID/users/:userID
{
"user": {
"created_at": "2022-04-29T15:18:14.116026Z",
"updated_at": "2022-04-29T15:18:49.229327Z",
"status": "active",
"id": "9DeEXvYBStnzwQcHFd6f558L",
"email": "anna@passage.id",
"phone": "",
"webauthn": false,
"webauthn_devices": [],
"last_login_at": "2022-04-29T15:18:49.228759Z",
"login_count": 1,
"user_metadata": {
"first_name": "anna",
"last_name": "pobletts"
}
}
}
User metadata can also be updated via the management API or SDK.
Node.js
const user = await passage.user.update(userId, {
userMetadata: {
first_name: 'hannah',
},
});
Learn more about the Node.js Complete SDK
Python
update = UpdateUserArgs(
user_metadata={
"first_name": "hannah",
},
)
user = passage.user.update(user_id, update)
Learn more about the Python Complete SDK
Go
update := passage.UpdateUserArgs{
UserMetadata: map[string]interface{}{
"first_name": "hannah",
},
}
user, err := psg.User.Update(userID, update)
Learn more about the Go Complete SDK
Ruby
user = passage.user.update(
user_id: user_id,
options: {
'user_metadata' => {
'first_name' => 'hannah',
},
})
Learn more about the Ruby Complete SDK
PHP
$update = new UpdateUserArgs([
'user_metadata' => 'hannah',
]);
$user = $passage->user->update($userId, $update);
Learn more about the PHP Complete SDK